What is an Exception?
An exception is an unwanted event in the program that interrupts the normal flow of the instructions. It is the unexpected outcome of the program. The major difference between the error and exception is that the exception can be handled by the program, unlike error.
Exception Handling in PHP
Exception handling is the process of handling the exception (run time errors) to induce the normal flow of the code execution. This is the set of actions we take to handle the unwanted behavior of the code. The main goal is to execute the program without any unexpected behavior and outcome of the code.
When an exception occurs in the program, It proceeds generally using these steps:
- The current code state is saved.
- The code implementation will switch to a custom exception handler function
- Then the handler resumes the execution from the saved code state, terminates the script execution / continues the execution from a different location in the code.
Need of Exception Handling in PHP
PHP provides different functionalities in exception handling. It can handle various runtime errors such as IOException, SQLException, ClassNotFoundException, and arithmetic exceptions.
The following keywords are used when we use exception handling in PHP- Try, catch, throw, finally.
- Try: It is the block of code in which an exception is present. This piece of code raises the exception. Try block should have a catch or finally block. It contains more than one catch block too.
- Throw: The throw keyword is used to symbolize the occurrence of a PHP exception Then we try to find a catch statement to handle this exception.
- Catch: This is the block of code that is executed when the try block throws an exception. It is responsible for handling the exception in the try block. It is always used with the try block.
- Finally: The PHP 5.5 version introduced the “finally” statement in PHP. The “finally” block can be used after a catch block or in place of catch blocks. “Finally “ term means that it will be executed no matter the exception is thrown or not. It generally executes the necessary parts of the program. We use it for many purposes like closing the files, closing the open databases connections, etc.
The Basic syntax which we use when handle the exceptions using try and catch is given below.
Multiple Exceptions– Multiple exceptions use multiple try-catch blocks for the implementation and execution of the code. It is used when you want to show the customized message or you wanted to perform some unique operation when the exception is thrown.
In the single program, multiple exceptions can be there like arithmetic exceptions, IO exceptions. We will help you to understand the concept of Multiple exceptions firstly by flowchart representation and then by executing the program.
Flowchart to Describe the working of Multiple Exceptions
Now here is an example for the multiple exceptions using the program.
We will implement the code that divides a number by the number in the denominator.
We have the chance to get the two types of exceptions by dividing the number by zero or by dividing the number by a negative number.
We can work with the multiple cases of exceptions
The code below shows the implementation.
Here we are writing the code when we divide a number by the denominator there are two chances of exceptions one is by dividing it to zero or by a negative number. In the program, we are using multiple catches to solve the exceptions.
Using multiple try-catch with multiple exception types
PHP supports the use of more than one nested catch block to handle their thrown exceptions. This provides us the permission to alter our code based on the type of exception thrown. This is useful for customizing how you display an error message. We can handle different types of exceptions using the catch blocks.
The syntax is given below:
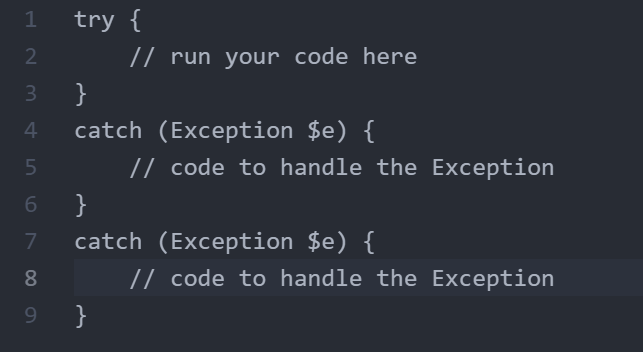
Use of try-catch-finally
Introduced in PHP version 5.5, Sometimes we can also use a “finally” section. Finally is useful in many cases it is not just limited to exception handling, it is used to perform the necessary tasks, and in the cases where the execution is independent of exceptions.
The “finally” block is always used when the try-catch block exits. It ensures that finally block is executed in any situation.
Example for using try-catch-finally:
Flowchart to tell you how this program works.
Explanation of the working of flowchart
Firstly we check If the exception occurs or not and if occurs it will be handled by the catch block. There are two chances-
- The exception will be handled
- The exception will not be handled.
If the exception is handled by the catch block then the “finally’’ block will be executed too.
If the exception is not handled, then also the “finally” block will be executed itself.
Conclusion of Exception Handling in PHP
The concept of exception is almost similar in all the languages. Exception Handling ensures the normal execution of the programs and we can handle it using try, catch, throw, and finally keywords in PHP.
Thanks For Reading
Read More on Aelum Blogs
Author: Rati Kumari Jha
Designation: Technical Content Writer